In this week’s Writer’s Room – a regular blog series of articles and tutorials written by technologists from within our Andela Community – Chesvic Hillary explains how you can consume the SOAP web service the Node.js way!
The word SOAP triggers goosebumps for both junior and experienced developers, yet it’s a web protocol that cannot be avoided because most legacy web services were implemented using SOAP.
While there are several ways to consume the SOAP web service, it usually leads to bad anti-pattern, like ‘callback hell’. Today’s post will help demystify SOAP and allow developers to consume a SOAP web service the same way they would normally consume a REST service.
What is SOAP?
SOAP is a messaging protocol that relies on application layer protocol, usually HTTP. This property makes it possible to consume SOAP in a REST fashion. To better understand SOAP, let’s take a look at its building blocks.

The building block tells us what is required for every SOAP request. We’ll take advantage of this by making the body dynamic and leaving other building blocks constant, as they will never change.
SOAP uses a WSDL file to formally describe what web services are available and what parameters are necessary to consume the service. It is like the REST API documentation, but written in XML (Who does that?).
Now, let us consume a public web service implemented with SOAP. We will consume this service the Node.js way.
Here is the WSDL for the public web service we will be using. We will be consuming the multiply operation of the web service.
STEP 1: Get SOAP Web service address from the WSDL
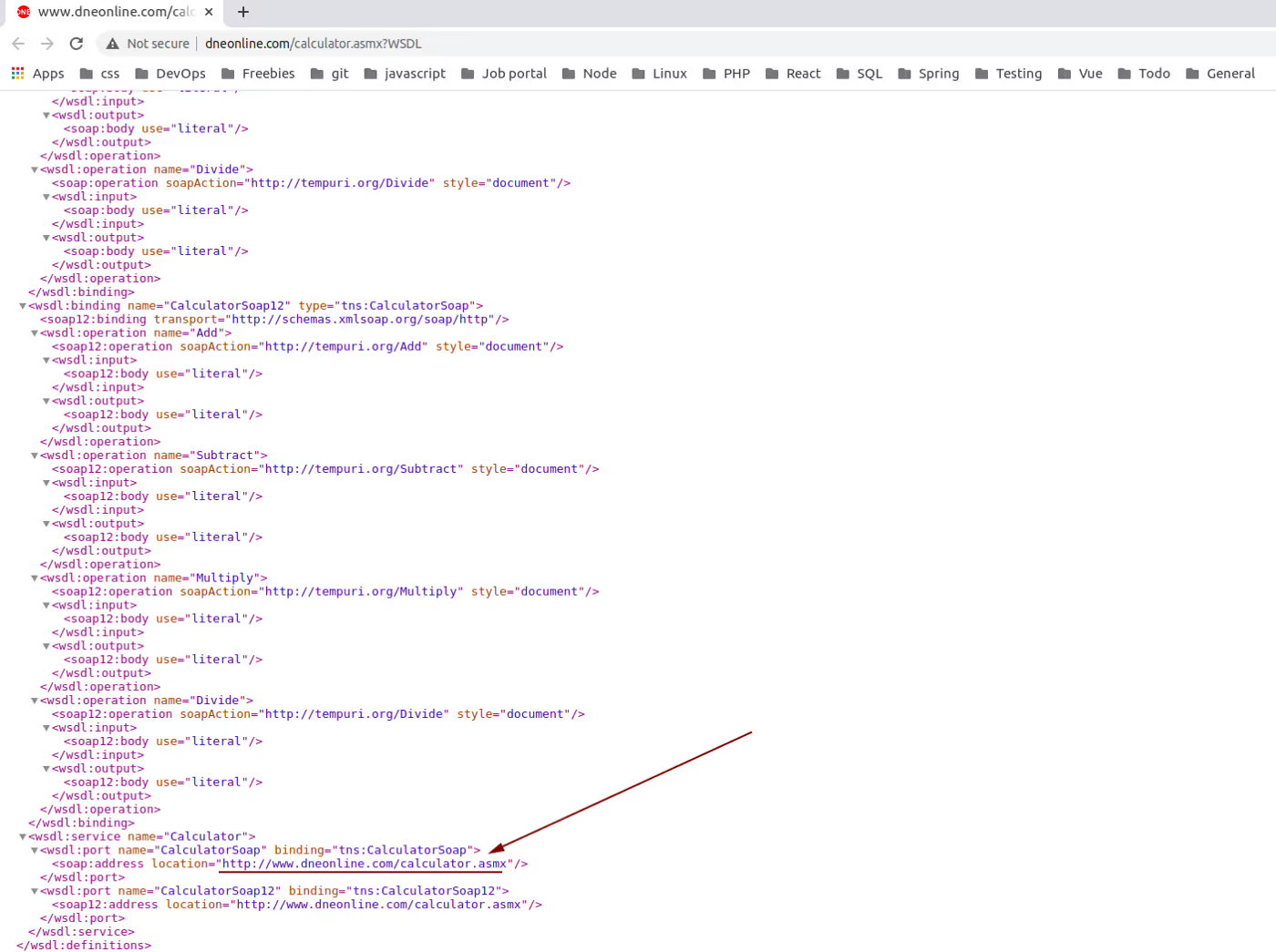
The SOAP address is equivalent to the REST Base URL. In our tutorial, it is the following: x
STEP 2: See supported operation of the web service
Type the SOAP address on your browser, which will open a list of supported operations on a web page.
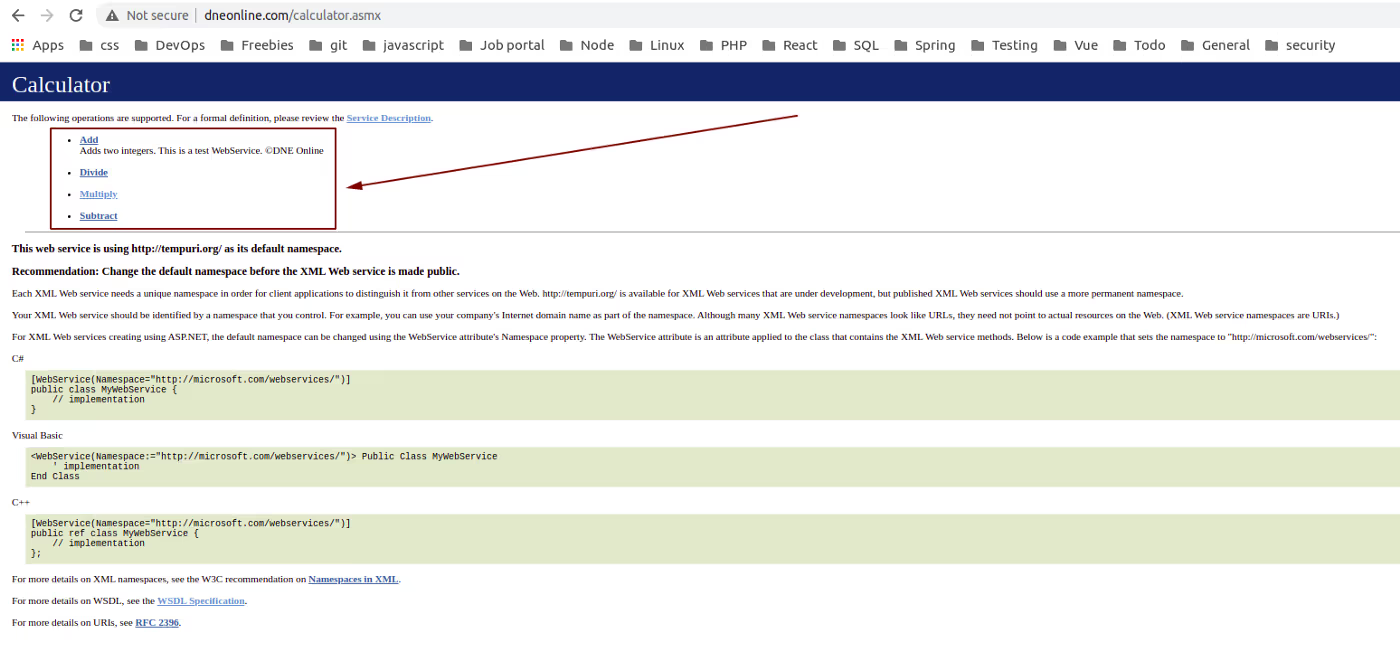
STEP 3: Review the request/response for an operation
Click on an operation to see the SOAP request and expected response.
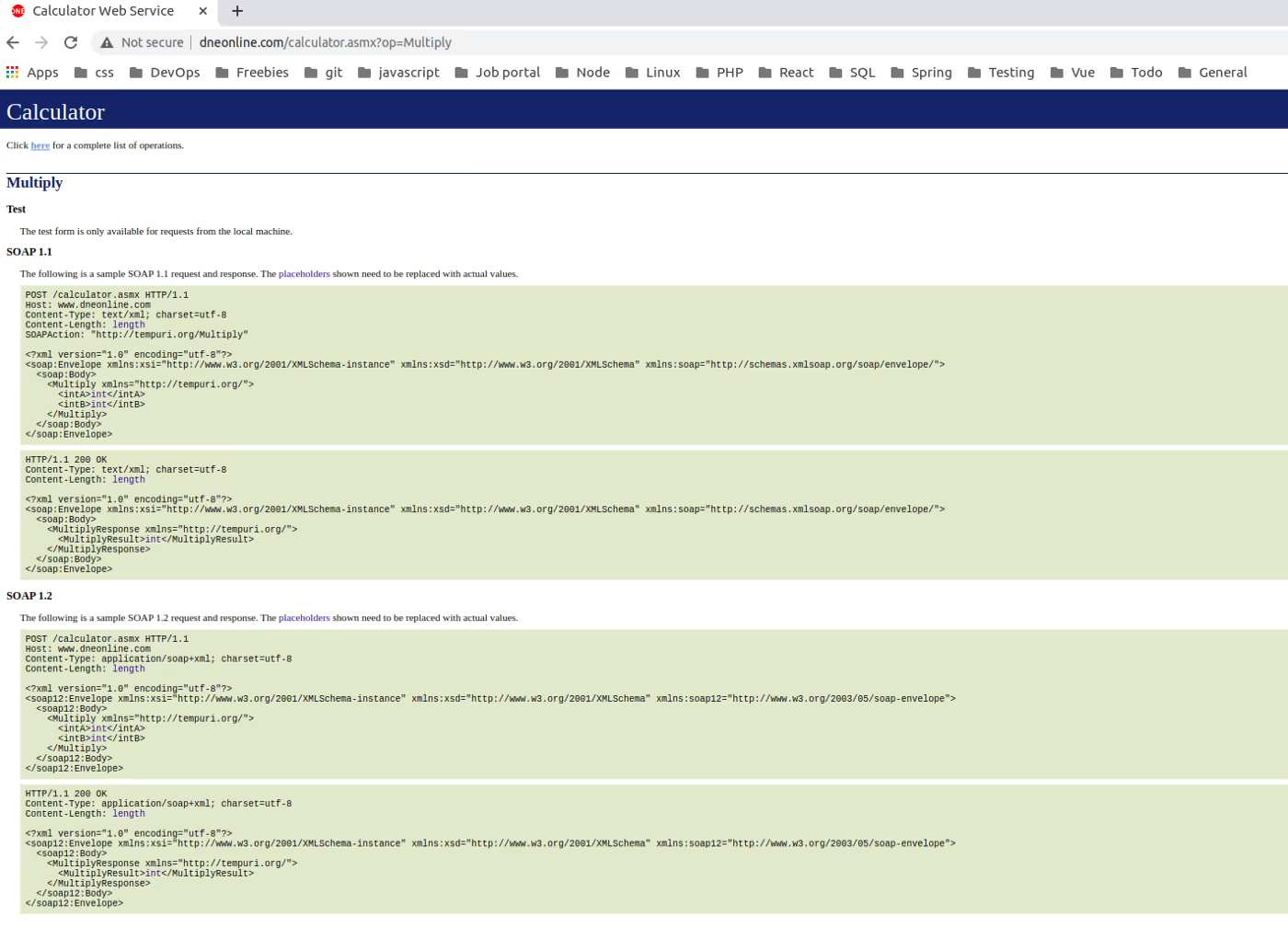

This gives a clue on how to send a request and get a response from any client. First, POST tells us this will be a HTTP POST verb. Right beside the verb we have the endpoint for that service: host point to where the service is hosted for the endpoint. Next, we have the headers. Notice that the soapAction and content-type will be sent via request headers. The content-length will be automatically calculated. The SOAP body for the request specify the required parameters.
Step 4: Test SOAP request using POSTMAN
With information received during step 3, we can easily test this service on POSTMAN (cool right?!).

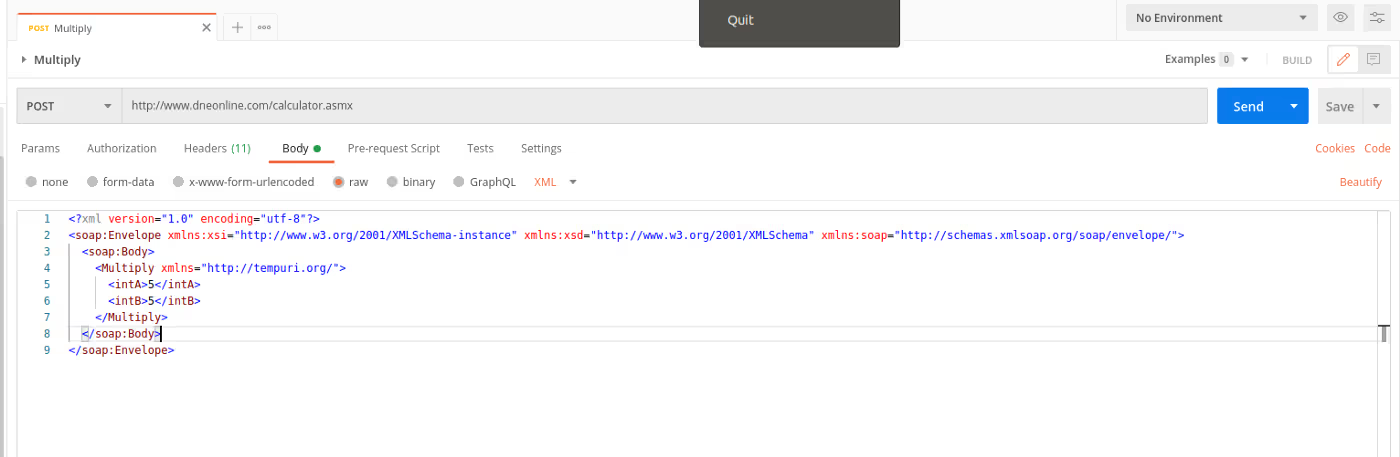
Notice that we change the INT placeholder to an actual value in this case. If we hit the send button, we get a successful response.

SOAP successful response
Step 5. Consume endpoint the Node.js way
Let’s create a utility function to convert JSON to XML, and vice versa, so we can comfortably work with JSON. Inside your node.js project, install the following package.
npm i xml2js jsontoxml
Then, add a utility class called parser.js to your application. See the following code below:

parser.js
See parser.js in github
It’s good practice to observe the separation of concerns when building your application. Now, let’s add another utility that conforms to the needs of the SOAP we are trying to consume.
Add a utility called formatter.js to the application.
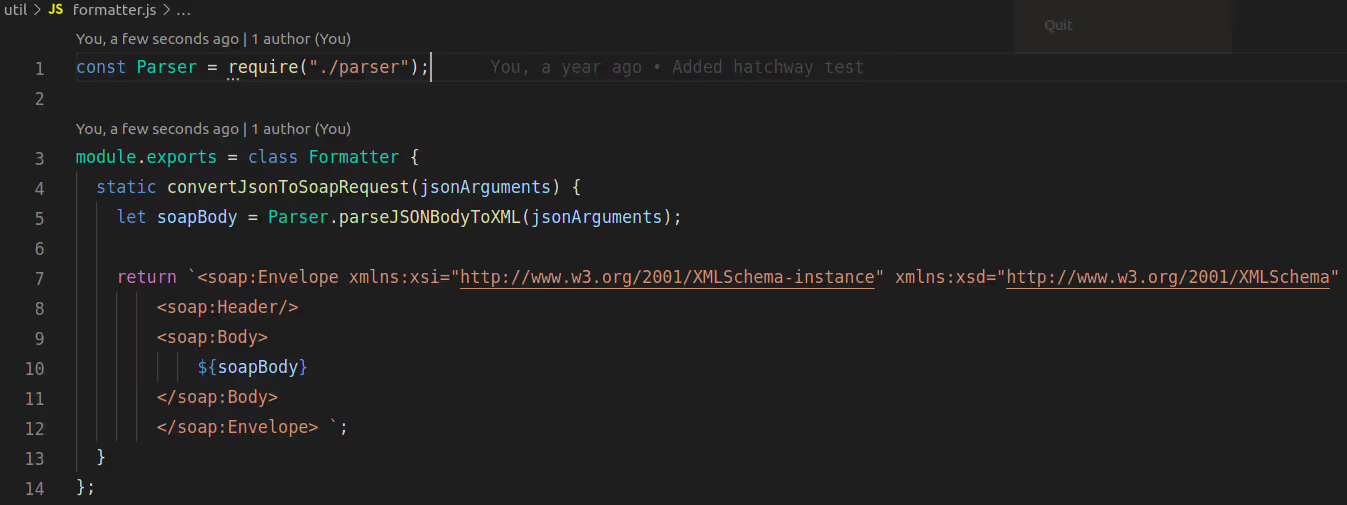
formatter.js
See formatter.js in github
Notice that we added the namespace xmlns=”http://tempuri.org/” to the Soap:Envelope, since it’s common in all operations for this particular SOAP.
Things are beginning to shape up! Next, let’s create a remote class that will make remote calls to the API service, just like we would normally do in REST application.
Add a class called remote.js to the application.

remote.js
See remote.js in github
Next, you just need to call the remote class from your service:
const Remote = require('./util/remote')
Remote.multipleTwoOperands(5,5);
You have your soap response, which was converted to JSON by your parser file.

That’s all there is to it. Keep hacking!
Want to be part of the Andela Community? Then join the Andela Talent Network!
With more than 175,000 technologists in our community, in over 90 countries, we’re committed to creating diverse remote engineering teams with the world’s top talent. And our network members enjoy being part of a talented community, through activities, benefits, collaboration, and virtual and in-person meetups.
All you need to do to join the Andela Talent Network is to follow our simple sign-up process.
Submit your details via our online application then…
- Complete an English fluency test – 15 minutes.
- Complete a technical assessment on your chosen skill (Python, Golang, etc.) – 1 hour.
- Meet with one of our Senior Developers for a technical interview – 1 hour.
Visit the Andela Talent Network sign-up page to find out more.
If you found this blog useful, check out our other resources for more essential insights!