No one wants to spend an entire day running a manual script on a thousand servers. Fortunately, many processes like this can be automated using Ansible.
For example, imagine you are given a task to run a command to detect the Timezone
on 1,000 servers. A quality engineer detected that some transactions are dated in the future, and the engineers suspected that some servers might have incorrect time zones. You are given a file that contains the IP addresses of 1,000 servers.
I will walk through completing this task by running an ad-hoc script to dynamically generate an inventory file using Ansible. All of this code is available on GitHub, and this tutorial assumes you already have an understanding of Python, Ansible and Bash.
Step 1
Establish a passwordless SSH connection with the target host. This will enable Ansible to securely log into the target host without having to input the password.
Step 2
Add the IP address to the file servers.txt
and ensure the IP address is valid and follows the format in the servers.txt
file.
Step 3
Extract the server’s IP address using Python to dynamically generate the inventory file:
#!/usr/bin/env python3
import re
import json
import os
# Get the current directory
current_directory = os.getcwd()
# Concatenate the current directory with the file name
server_file = os.path.join(current_directory, 'servers.txt')
def read_servers_file(server_file):
"""Reads the server file and extracts the IP addresses."""
ips = []
with open(server_file, 'r') as f:
lines = f.readlines()
for line in lines:
if 'ip_address=' in line:
match = re.search(r'ip_address=([\d\.]+)', line)
if match:
ips.append(match.group(1))
return ips
def generate_inventory(ips):
"""Generates the inventory in JSON format."""
inventory = {
'_meta': {
'hostvars': {}
},
'all': {
'hosts': ips
}
}
return inventory
def main():
"""Main function."""
ips = read_servers_file(server_file)
inventory = generate_inventory(ips)
print(json.dumps(inventory))
if __name__ == '__main__':
main()
Step 4
Save the following Ansible playbook as ansible-playbook.yml
:
---
- name: Extract ctlplane IP addresses and run command on servers
hosts: all
gather_facts: yes
become: yes
remote_user: ec2-user #change this to the remote user
tasks:
- name: Run command on servers and save output locally
ansible.builtin.shell: "date"
register: command_output
run_once: yes
- name: Debug command output
ansible.builtin.debug:
msg: "{{ command_output.stdout }}"
- name: Create your local file on master node
ansible.builtin.file:
path: "report.txt"
state: touch
mode: '0644'
delegate_to: localhost
become: no
- name: Create report.txt file or append to existing file
ansible.builtin.lineinfile:
path: "report.txt"
line: "{{ item }} - {{ command_output.stdout }}"
loop: "{{ ansible_play_batch }}"
delegate_to: localhost
become: no
Step 5
Run the Ansible playbook, which will run a command date
on the target servers and display the output in a file called report.txt
, with:
ansible-playbook -i dynamic_inventory.py ansible-playbook.yml
Step 6
Your output should look similar to this screenshot.
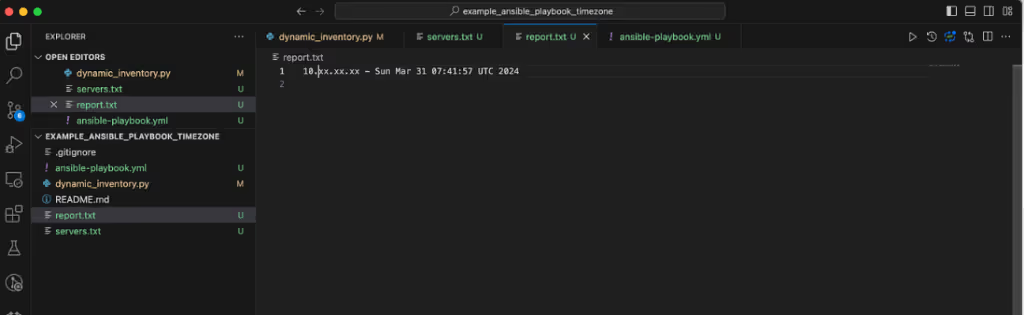
Conclusion
Ansible simplifies complex tasks, such as instance infrastructure provisioning, configuration management and software deployment across a large-scale environment. You can find the full code for this tutorial on GitHub.
About the author: Adetokunbo Ige
Adetokunbo Ige is a technologist for Andela. A seasoned Platform Engineer and a Certified ISO 22301 Lead Implementer in Business Continuity, he brings a wealth of experience in software engineering, enterprise application management, server infrastructure management, database management, incident management, and cloud engineering. He holds a B.Sc in Computer Science from Babcock University and an M.Sc in Business Information Technology from Middlesex University, where he graduated with distinction. His technical proficiencies span various programming languages and tools including SQL Server, Oracle, MySQL, Docker, Kubernetes, and numerous scripting languages.