Concurrency in Swift
In this Writer's Room blog, Andela community member Muhammad Afham provides a bite-sized dive into concurrency in Swift, an important factor in the realm of iOS development, and offers different ways to achieve concurrency.
What is concurrency?
- The ability to run multiple tasks or processes simultaneously.
- Makes applications more efficient, responsive, and scalable.
- Allows applications to make the most of available hardware resources.
What is a thread?
A thread is a lightweight, independent unit of execution within a process. Threads enable concurrent execution within a single process and allow a program to perform multiple tasks simultaneously.
Problems
- Race conditions: When two or more threads access shared resources concurrently, they can create race conditions, where the final result depends on the order in which the threads execute. This can lead to unpredictable behavior and bugs that are difficult to reproduce and debug.
- Deadlocks: Deadlocks occur when two or more threads are blocked, waiting for resources that are held by other threads, creating a situation where none of the threads can proceed. Deadlocks can cause the program to become unresponsive or crash.
How we can achieve concurrency in Swift?
- Thread ( Class )
- Grand Central Dispatch (GCD)
- OperationQueue
- Async-Await ( Swift 5.5 )
Types of queues in Swift
Here are the types of queues in Swift, along with a brief description of each:
- Global queues (Non-UI Tasks : Global queues are concurrent queues that are provided by the system. There are four global queues, each with a different priority level. They are used for performing background tasks, such as network operations or file I/O.
- Main thread queue (UI Tasks): The main thread queue is a serial queue that is synonymous with the main queue. It is used for executing tasks on the main thread, which is the thread that is responsible for the user interface.
- Custom queues: Custom queues are private queues that you create yourself using the DispatchQueue class. You can specify whether a custom queue is serial or concurrent depending on your needs.
1. Dispatching a task on a serial queue:

2. Dispatching a task on a concurrent queue:

3. Dispatching a task on the main queue:

4. Dispatching a task on a global queue:

5. Operation Queue ( Concurrent Queue)

- Dispatching multiple tasks concurrently using DispatchGroup:
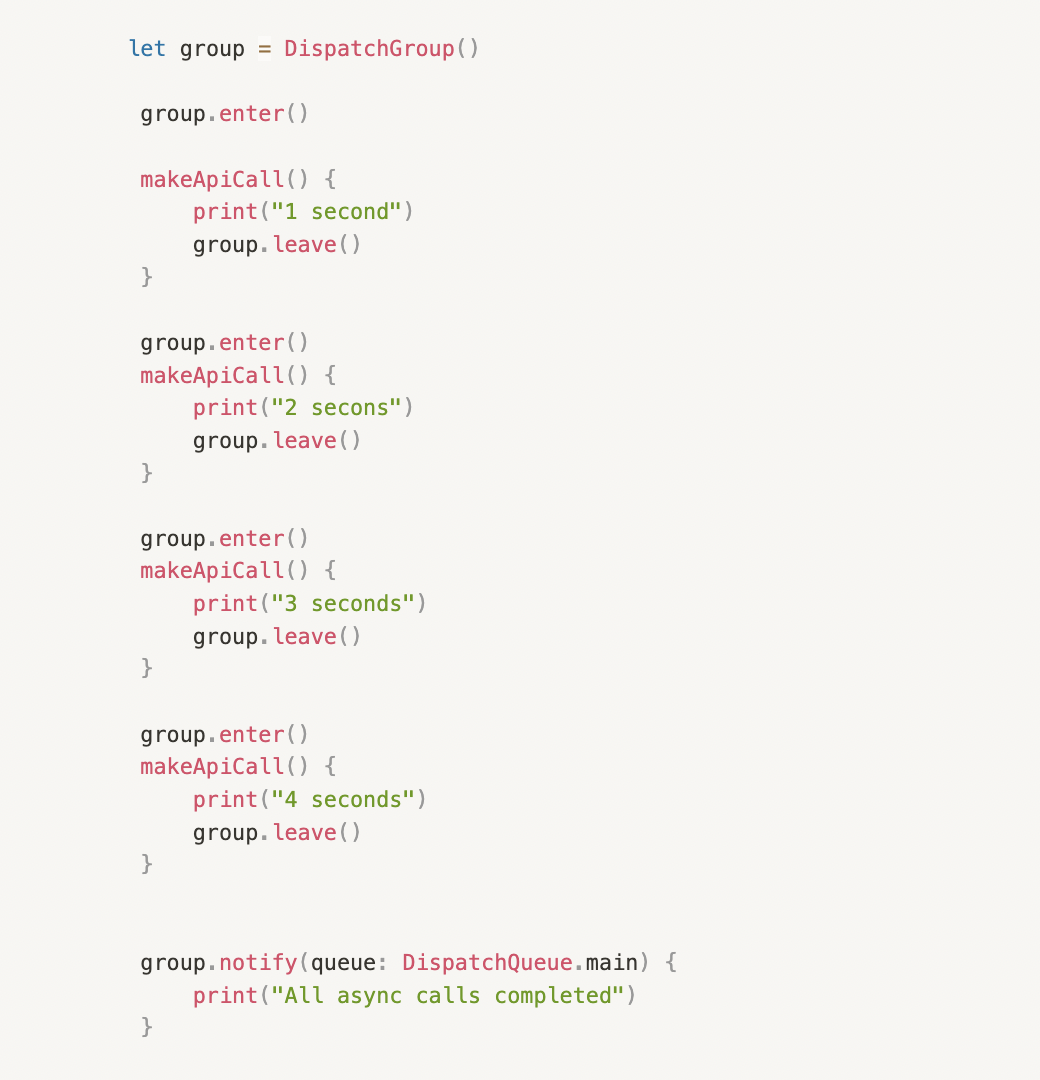
Difference b/w “serial” and “concurrent” with async?
Serial queue
- Executes tasks one at a time.
- Tasks are executed in the order they are added to the queue.
- A task won't start until all tasks before it in the queue have been completed.
- Useful when task order is important (e.g. database transactions, updating UI).
Concurrent queue
- Can execute multiple tasks simultaneously.
- Tasks may start immediately, even if other tasks are still running.
- Useful when maximizing performance and throughput is important (e.g. computations, network operations).
Summary
- Serial vs concurrent queue (Related to tasks execution in parallel or sequence)
- Sync vs async (Related to calling thread locking)
Queues:
- Queues are used to manage tasks in Grand Central Dispatch.
- There are two types of queues: serial and concurrent.
- Serial queues execute tasks one at a time, in the order, they are added to the queue.
- Concurrent queues can execute multiple tasks simultaneously.
- The system provides four global concurrent queues with different quality of service (QoS) levels: userInteractive, userInitiated, utility, and background.
- Custom queues can also be created to control task execution orders or isolate tasks.
Dispatch groups:
- Dispatch groups allow you to group tasks and wait for them to complete.
- Tasks can be added to a group by calling group.enter() before the task and group.leave() after the task is complete.
- group.notify { // all tasks are completed } can be used to execute a completion handler after all tasks in the group are complete.
Resources
https://developer.apple.com/tutorials/app-dev-training/adopting-swift-concurrency
https://developer.apple.com/tutorials/app-dev-training/modernizing-asynchronous-code
https://www.kodeco.com/28540615-grand-central-dispatch-tutorial-for-swift-5-part-1-2
Related posts
The latest articles from Andela.
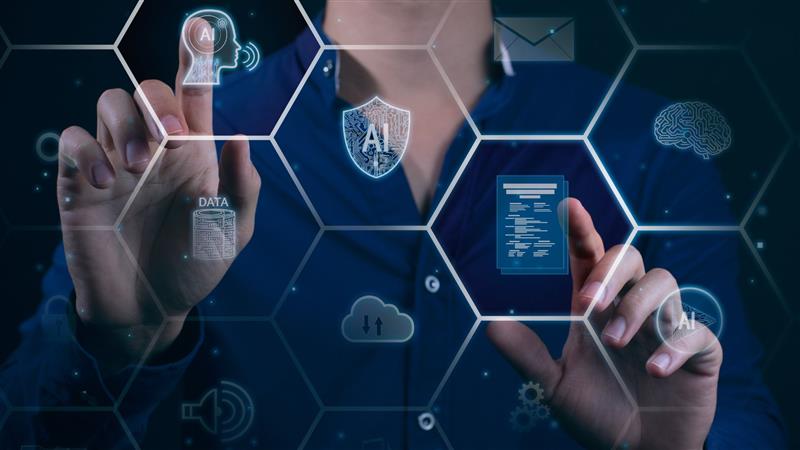
What GPT-4o and Gemini releases mean for AI
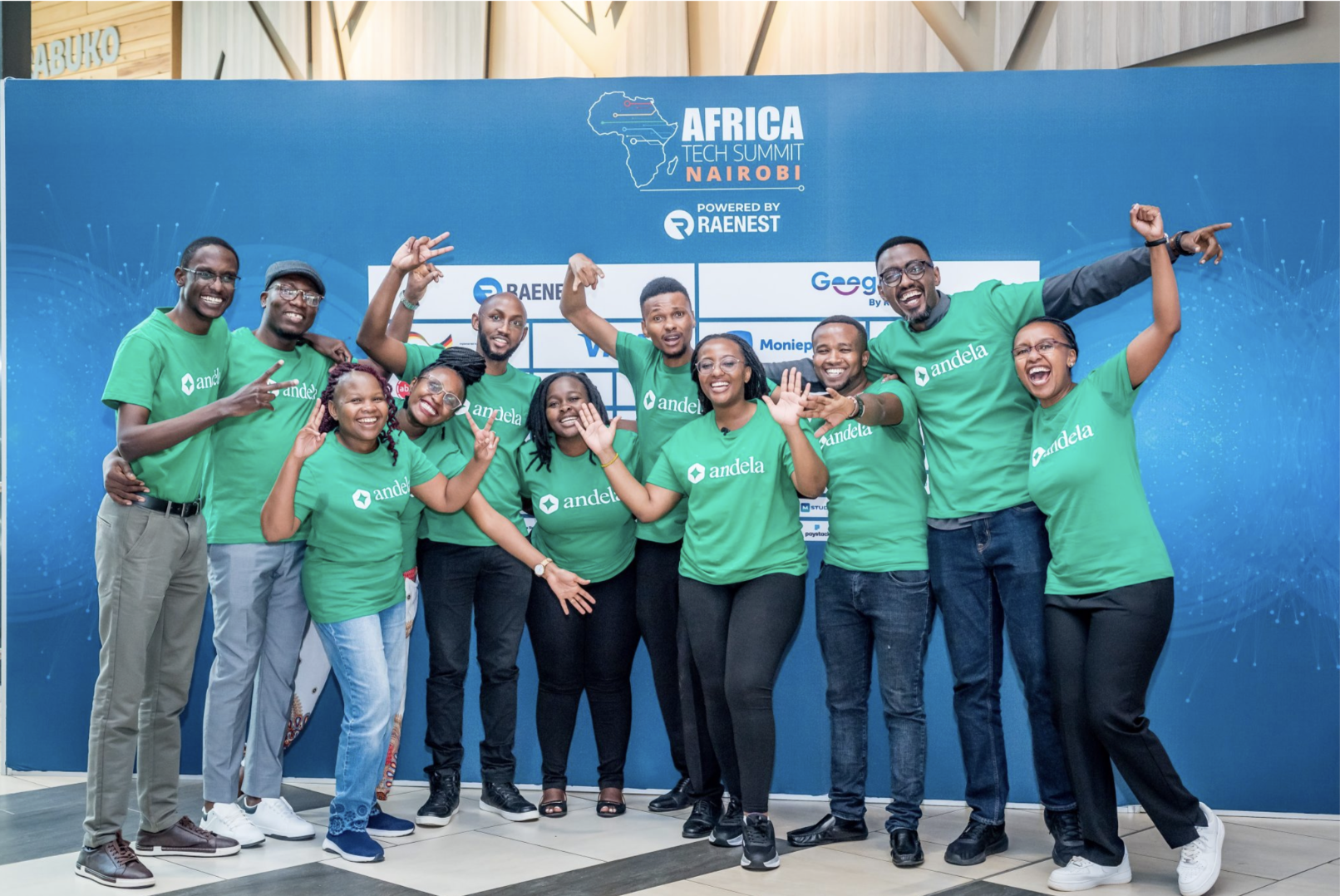
How Africa’s Tech Talent is Making an Impact Across the Continent
.jpg)
Cancel Asynchronous React App Requests with AbortController
We have a 96%+
talent match success rate.
The Andela Talent Operating Platform provides transparency to talent profiles and assessment before hiring. AI-driven algorithms match the right talent for the job.
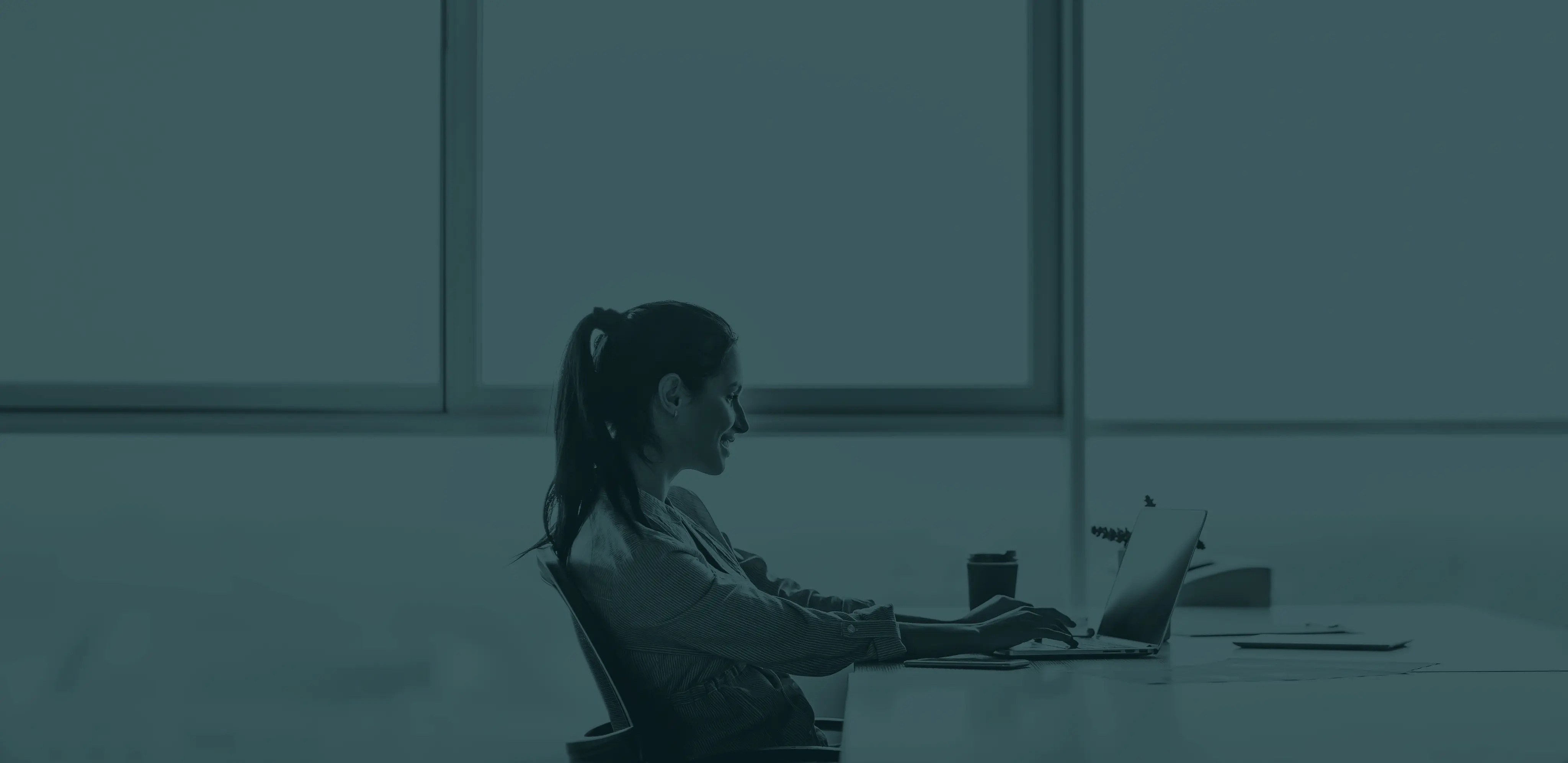