Cancel Asynchronous React App Requests with AbortController
When developing web applications, it is common practice to send asynchronous requests to a backend server. However, there are times when these requests become redundant and need to be canceled, such as when a user accidentally initiates a large file download and decides to stop it.
Offering a mechanism for users to cancel unintended requests is beneficial, and the AbortController web API is an effective way to do so in React applications.
What Is AbortController?
AbortController is a web API that can abort one or web requests as and when needed. This is particularly useful for managing asynchronous tasks, such as fetch
requests in web development. AbortController creates a signal that can be passed to the Fetch API or other APIs that support terminating web requests.
You can call the abort
method on the AbortController instance when you want to stop the ongoing operation. This signals to the request that it should be canceled.
AbortController’s benefits include:
- Improved user experience: Canceling unneeded requests can reduce the load on your server and alleviate network congestion. This can enhance the overall performance of your application and improve user experience.
- Help solve race conditions: A race condition occurs when two or more processes try to access or modify a shared resource simultaneously, resulting in an unpredictable output. The outcome of the process depends on the order they executed, and this order can vary depending on factors such as network speed, device performance or server load. AbortController can help mitigate race conditions by allowing you to cancel a request that is no longer needed, thereby preventing unnecessary collisions between different processes.
How to Use AbortController in React
I will demonstrate how AbortController can be used to solve the race condition problem with the Axios HTTP client for Node.js.
Set up AbortController
The following code creates a React component with two buttons: one gets a random picture using the random user generator API and another cancels the request. It also conditionally renders a generated image from the request. Furthermore, it includes a pictureUrl
state that stores the URL of the picture generated.

Add API Requests
The following code imports Axios, which enables making the API request to the random user generator API. It also includes an onClick
event listener that calls the getPicture
function. This function requests the endpoint and sets the image generated to the pictureUrl
state.
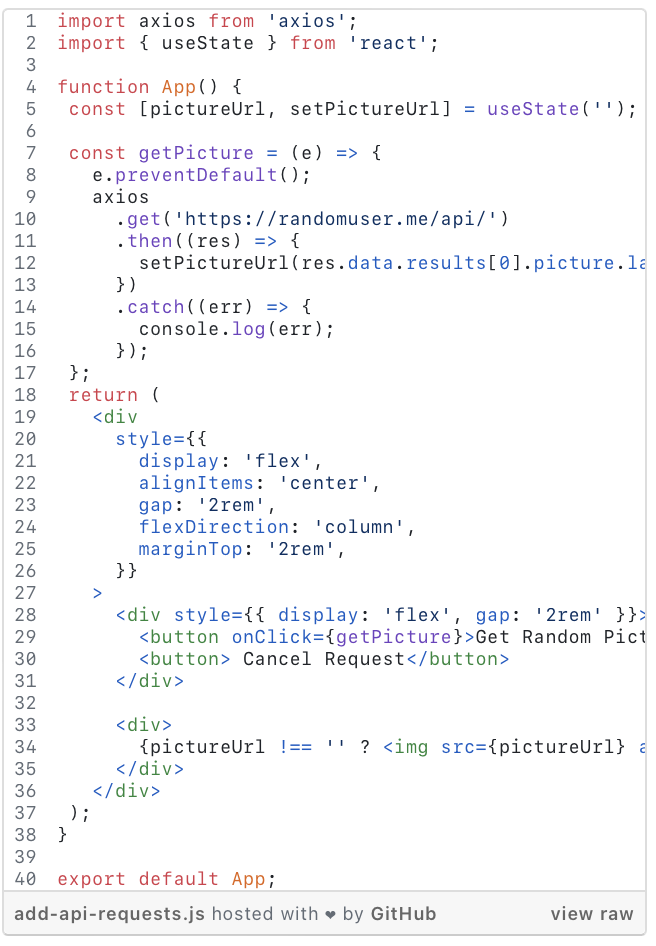

Canceling the Request
The following code creates a ref for the abortController
and initializes it with an empty string. This prevents React from rerendering the component when the ref value changes. For each API request, it creates a new abortController
and passes its signal property (a read-only property of the abortController
that can be used to communicate with the request) to the request. This ensures each request has its own controller object. It also adds an onClick
event listener for the cancel button, which calls the controller’s abort()
method to cancel the request.

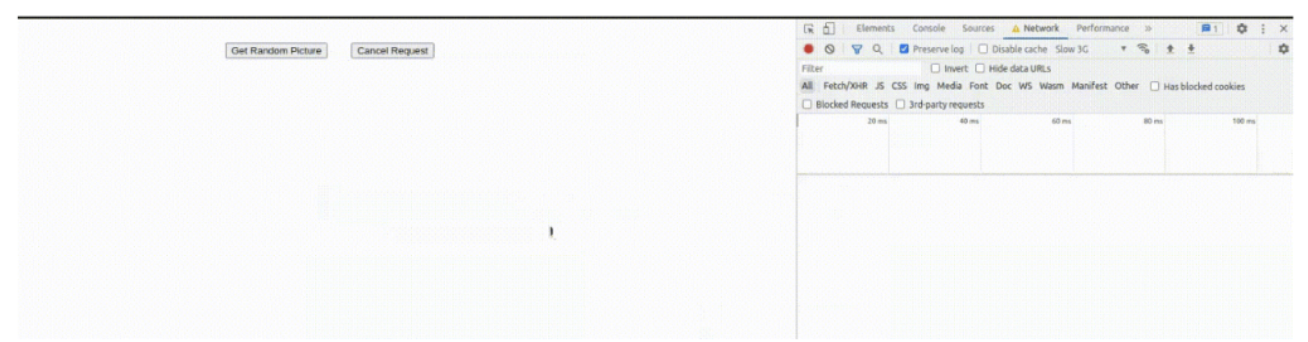
Conclusion
AbortController is a powerful tool for managing asynchronous requests in React applications and can help improve application performance and reliability.
If you are working with asynchronous requests in your React application, consider scenarios where you would want to cancel requests and use the AbortController API to do so effectively.
About the author: Adam Labaran
Adam Labaran is a software engineer and a member of the Andela Talent Network, a private global marketplace for digital talent. With extensive experience in building software, he has developed and highly scalable RESTful APIs using technologies such as Node.js (Express and Nest.js) and Python (Flask). Adam loves creating incredible user experiences while seeking to bring his unique visions to life.
Related posts
The latest articles from Andela.
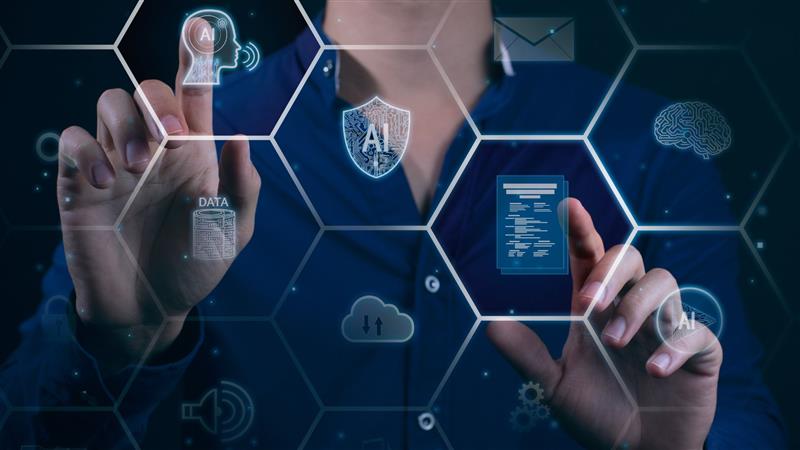
What GPT-4o and Gemini releases mean for AI
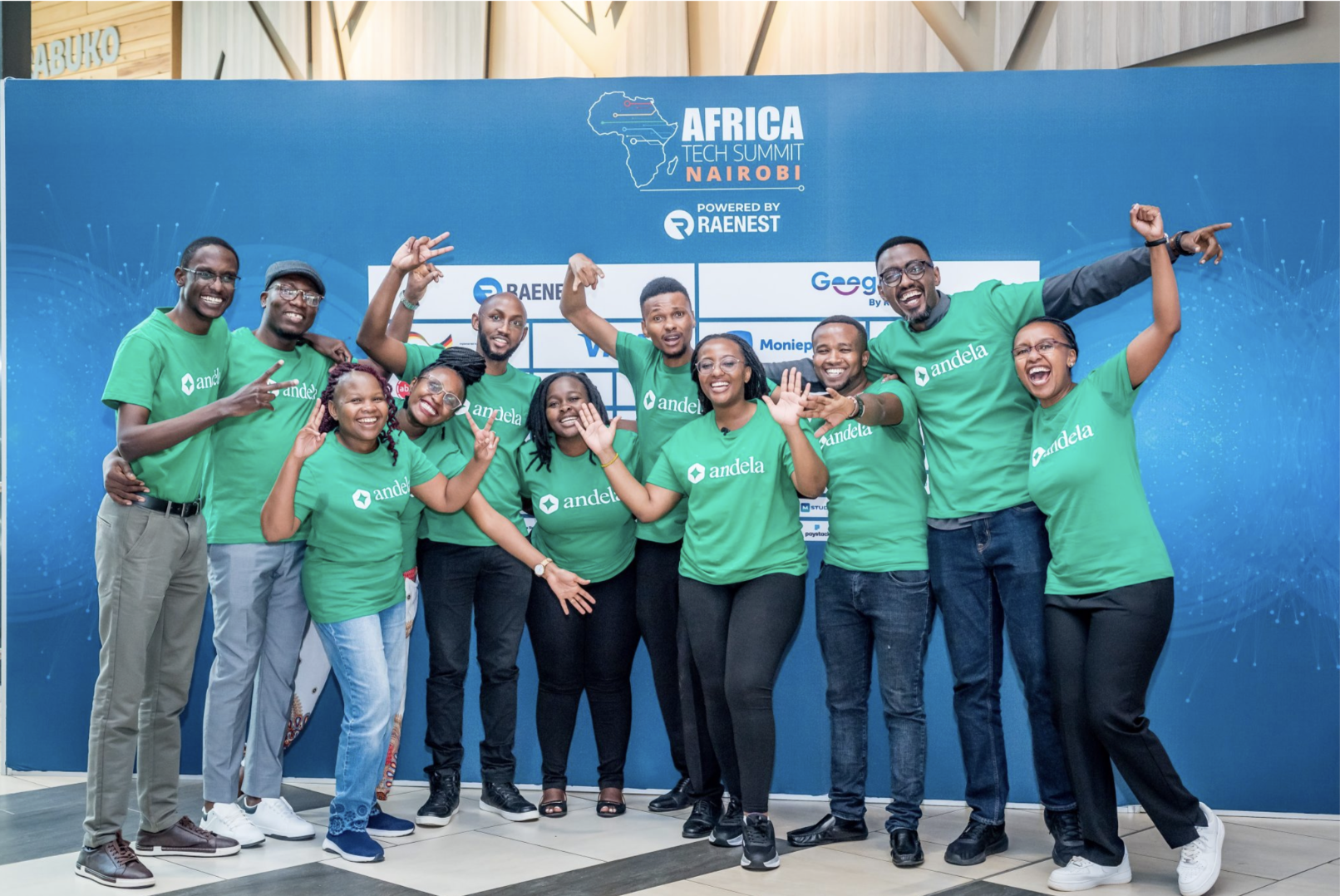
How Africa’s Tech Talent is Making an Impact Across the Continent
.jpg)
Cancel Asynchronous React App Requests with AbortController
We have a 96%+
talent match success rate.
The Andela Talent Operating Platform provides transparency to talent profiles and assessment before hiring. AI-driven algorithms match the right talent for the job.
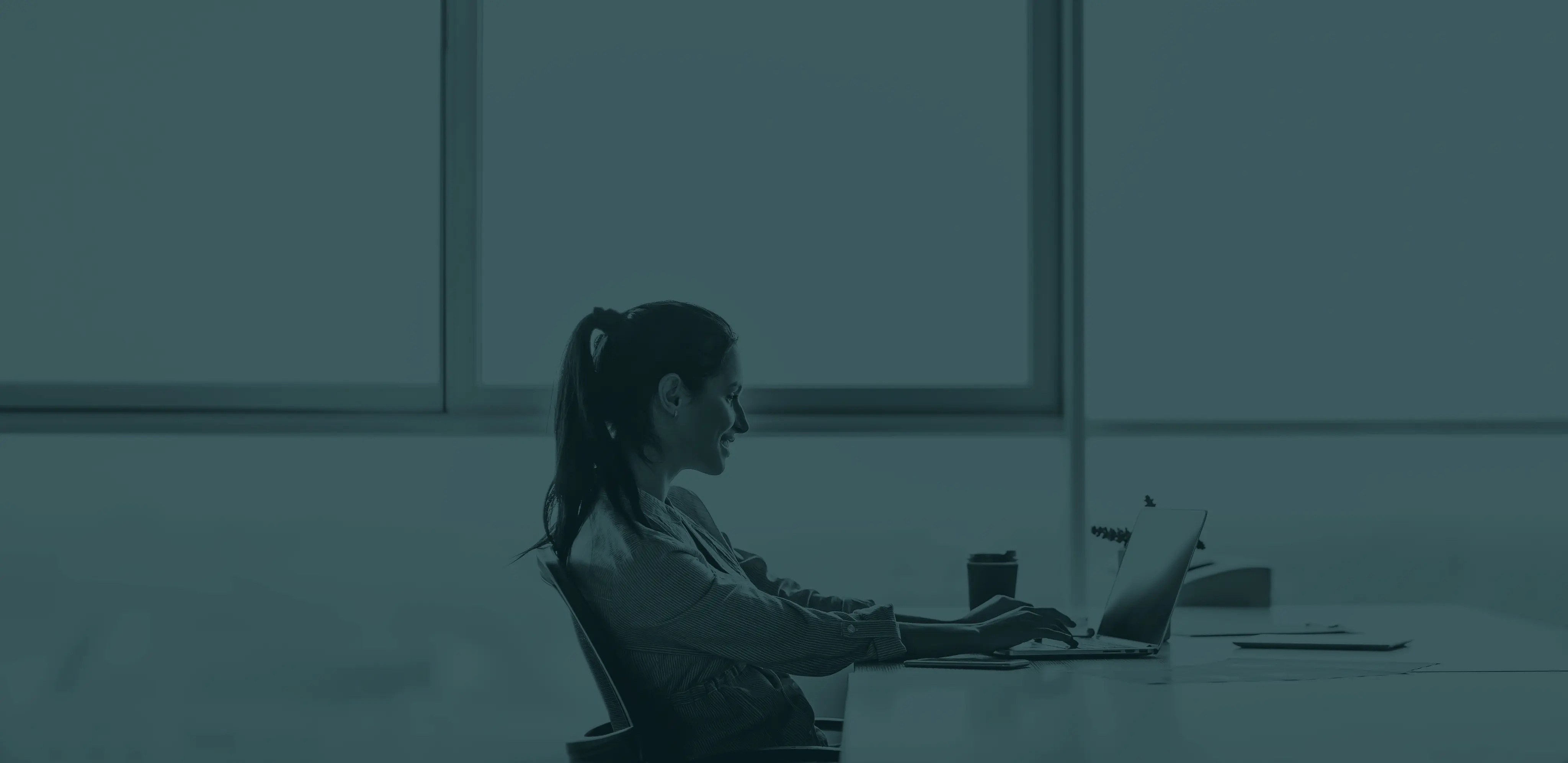