Javascript Convert to Boolean Using !! (double bang) Operator
Every value has an associated boolean, true or false, value in JS. For example, a null value has an associated boolean value of false. A string value, such as abc has an associated boolean value of true.Values that are associated with boolean true are said to be truthy. Values that are associated with boolean false values are said to be falsy.A full list of truthy values can be found here:TruthyA full list of falsy values can be found here:FalsyA single “!” symbol in javascript, also called a “bang”, is the logical “not” operator. If you place this operator in front of a boolean value, it will reverse the value, returning the opposite.[pastacode lang="javascript" manual="!true%3B%20%2F%2F%20Returns%20false.%0A!false%3B%20%2F%2F%20Returns%20true." message="" highlight="" provider="manual"/]If a bang (“!”) returns the opposite truthy value, what would a bang executed twice on a value do?[pastacode lang="javascript" manual="const%20x%20%3D%20true%3B%20%2F%2F%20Associated%20with%20true.%0Aconst%20a%20%3D%20!x%3B%20%2F%2F%20The%20returns%20the%20opposite%20of%20'x'%2C%20false.%0Aconst%20b%20%3D%20!a%3B%20%2F%2F%20The%20!%20takes%20value%20'a'%20of%20false%20and%20reverses%20it%20back%20to%20true.%0A%0A!!true%20%2F%2F%20Evaluates%20to%20true.%0A!!false%20%2F%2F%20Evaluates%20to%20false." message="" highlight="" provider="manual"/]So, running a bang twice determines the opposite of value, and then returns the opposite of that.With non-boolean values, it is kind of cool to see what the double bang does:[pastacode lang="javascript" manual="const%20x%20%3D%20'abc'%3B%20%2F%2F%20Associated%20with%20true.%0Aconst%20a%20%3D%20!x%3B%20%2F%2F%20The%20!%20determines%20x%20is%20associated%20with%20true%20and%20returns%20the%20opposite%2C%20false.%0Aconst%20b%20%3D%20!a%3B%20%2F%2F%20The%20!%20takes%20value%20'a'%20of%20false%20and%20reverses%20it%20to%20true.%0A%0A!!'abc'%20%2F%2F%20Evaluates%20to%20true.%0A!!null%20%2F%2F%20Evaluates%20to%20false.%0A%0A" message="" highlight="" provider="manual"/]Knowing the associated boolean value of any given value is helpful if you want to quickly reduce a value to a boolean:[pastacode lang="javascript" manual="function%20BankAccount(cash)%20%7B%0Athis.cash%20%3D%20cash%3B%0Athis.hasMoney%20%3D%20!!cash%3B%0A%7D%0Avar%20account%20%3D%20new%20BankAccount(100.50)%3B%0Aconsole.log(account.cash)%3B%20%2F%2F%20100.50%0Aconsole.log(account.hasMoney)%3B%20%2F%2F%20true%0A%0Avar%20emptyAccount%20%3D%20new%20BankAccount(0)%3B%0Aconsole.log(emptyAccount.cash)%3B%20%2F%2F%200%0Aconsole.log(emptyAccount.hasMoney)%3B%20%2F%2F%20false" message="" highlight="" provider="manual"/]In this case, if an account.cash value is greater than zero, the account.hasMoney will be true.Let’s see another example:[pastacode lang="javascript" manual="const%20userA%20%3D%20getUser('existingUser')%3B%20%2F%2F%20%7B%20name%3A%20Abel%2C%20status%3A%20'lit'%20%7D%0Aconst%20userB%20%3D%20getUser('nonExistingUser')%3B%20%2F%2F%20null%0A%0Aconst%20userAExists%20%3D%20!!userA%3B%20%2F%2F%20true%0Aconst%20userBExists%20%3D%20!!userB%3B%20%2F%2F%20false" message="" highlight="" provider="manual"/]
Related posts
The latest articles from Andela.
.jpg)
Customer-obsessed? 4 Steps to improve your culture
.jpg)
How to Build a RAG-Powered LLM Chat App with ChromaDB and Python
.jpg)
Navigating the future of work with generative AI and stellar UX design
We have a 96%+
talent match success rate.
The Andela Talent Operating Platform provides transparency to talent profiles and assessment before hiring. AI-driven algorithms match the right talent for the job.
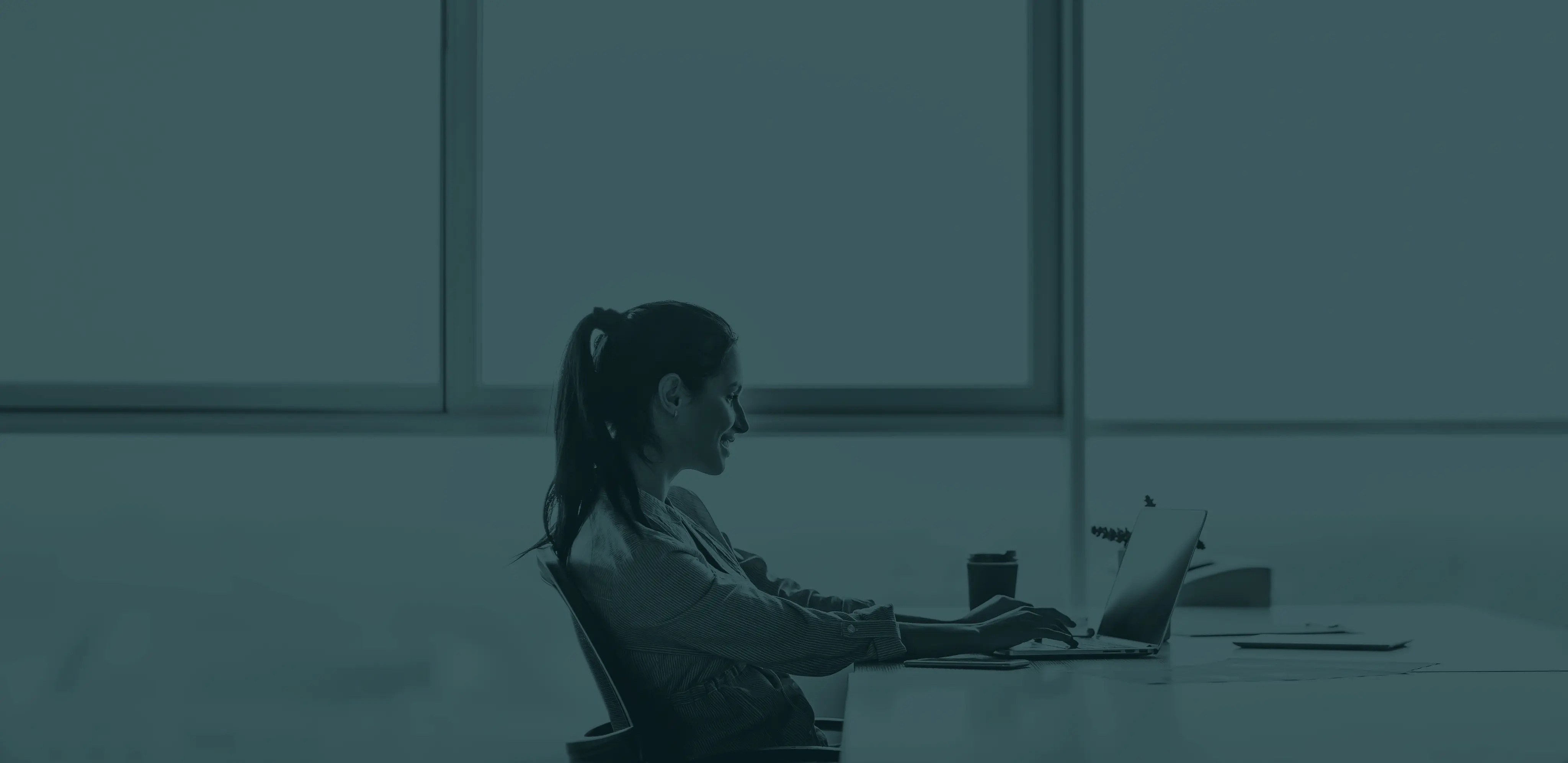